To convert a QBCore script to use the Ox (Ox-MySQL, Ox-Lib, etc.) framework, you’ll need to follow these general steps. This process assumes you have a basic understanding of both QBCore and Ox frameworks.
1. Setting Up Ox
First, ensure you have the Ox resources installed on your server. You can find Ox resources on GitHub or other repositories. Common Ox resources include:
- Ox-MySQL
- Ox-Lib
- Ox-Target
2. Updating Dependencies
Update the fxmanifest.lua
of your script to include Ox dependencies.
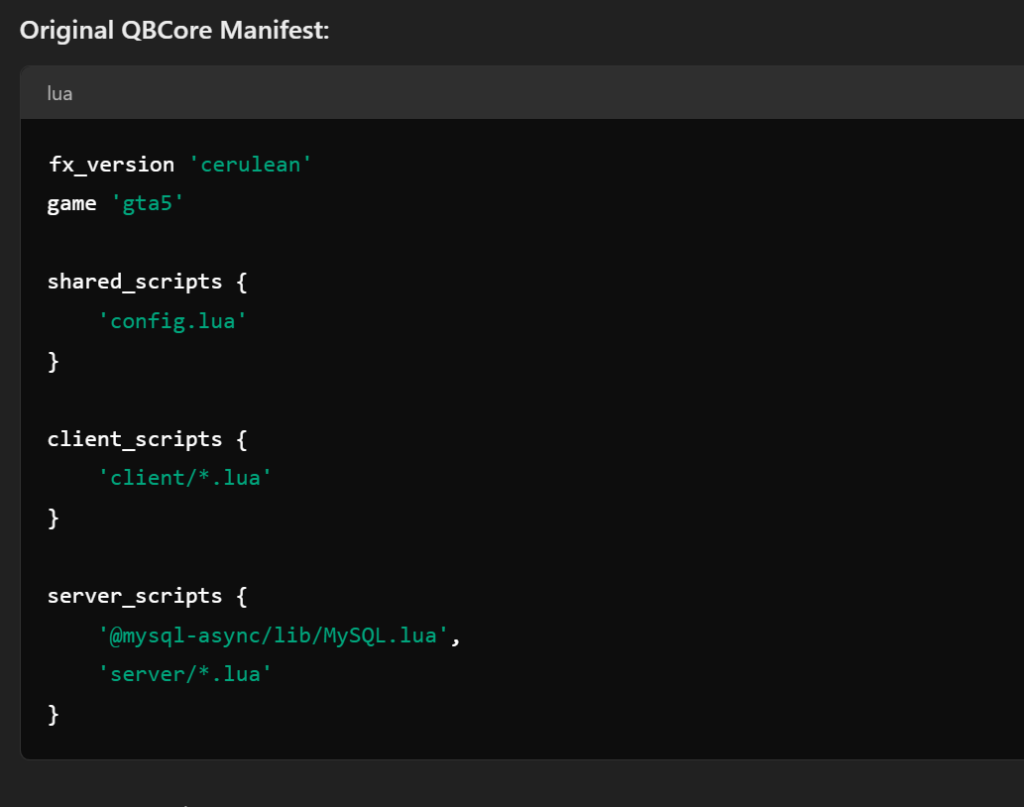
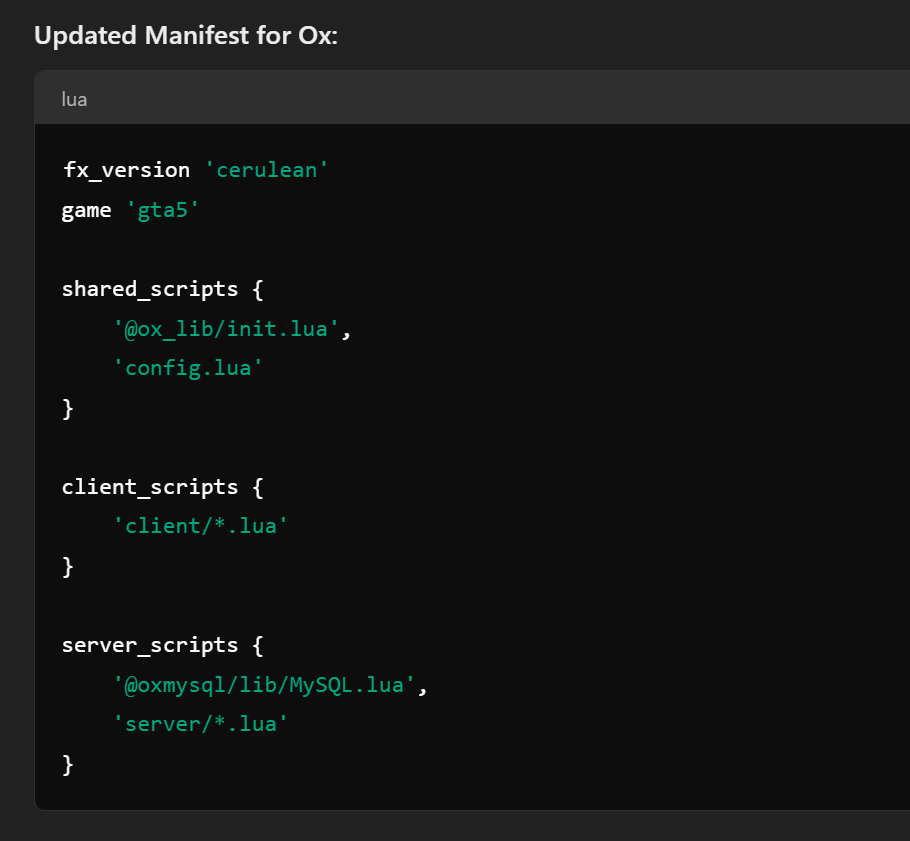
3. Database Interaction
Replace mysql-async
functions with oxmysql
functions. Here are some common conversions:
QBCore with mysql-async:
MySQL.Async.fetchAll('SELECT * FROM users WHERE id = @id', {['@id'] = id}, function(result)
-- Do something with the result
end)
Ox-MySQL:
local result = MySQL.query.await('SELECT * FROM users WHERE id = ?', { id })
-- Do something with the result
4. Event Handling
If your QBCore script uses QBCore-specific events, you need to replace them with Ox equivalents.
QBCore:
RegisterNetEvent('QBCore:Server:PlayerLoaded', function(playerId)
-- Your code here
end)
OX:
AddEventHandler('ox:playerLoaded', function(playerId)
-- Your code here
end)
5. UI and Targeting
For UI and targeting, replace qb-target
with ox-target
.
QBCore Target:
luaCopy codeexports['qb-target']:AddBoxZone("myTarget", vector3(0.0, 0.0, 0.0), 1, 1, {
name = "myTarget",
heading = 0,
debugPoly = false,
minZ = 0,
maxZ = 1
}, {
options = {
{
event = "myEvent",
icon = "fas fa-circle",
label = "My Target"
}
},
distance = 1.5
})
Ox-Target:
luaCopy codeexports.ox_target:addBoxZone({
coords = vec3(0.0, 0.0, 0.0),
size = vec3(1, 1, 1),
rotation = 0,
debug = false,
options = {
{
event = "myEvent",
icon = "fas fa-circle",
label = "My Target"
}
}
})
6. Updating Other Functions
Ensure that any other QBCore-specific functions or utilities are replaced with Ox equivalents. This includes functions for player management, money handling, inventory, etc.
7. Testing
After converting the script, thoroughly test it to ensure all functionalities work as expected. Check the server console and client console for any errors and debug as necessary.
Example Conversion
Original QBCore Script:
luaCopy codeQBCore.Functions.CreateCallback('myCallback', function(source, cb, data)
MySQL.Async.fetchAll('SELECT * FROM users WHERE id = @id', {['@id'] = data.id}, function(result)
cb(result)
end)
end)
Converted Ox Script:
luaCopy codelib.callback.register('myCallback', function(source, data)
local result = MySQL.query.await('SELECT * FROM users WHERE id = ?', { data.id })
return result
end)
Converting a QBCore script to use Ox framework involves updating dependencies, replacing database interactions, changing event handlers, updating UI and targeting logic, and ensuring all other QBCore-specific functionalities are converted to their Ox equivalents. Testing thoroughly after conversion is crucial to ensure the script works as intended
Additional Tips
- Break Down the Script: Divide the script into smaller, manageable components to simplify the conversion process.
- Leverage Community Resources: Search for existing conversions or guides that can provide valuable insights.
- Consider OX Alternatives: If certain functionalities are challenging to replicate, explore alternative OX resources or libraries.
- Seek Expert Help: If you encounter significant difficulties, consider consulting with experienced developers or the OX community.